打卡leetcode每日一题
Num322 零钱兑换
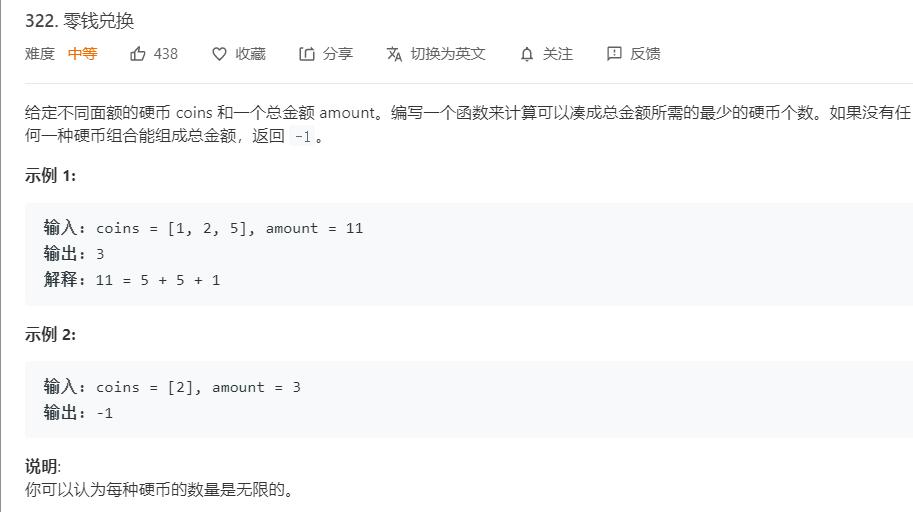
1 |
|
2 | int res =Integer.MAX_VALUE,count = 0; |
3 | public int coinChange(int[] coins, int amount) { |
4 | if(amount==0) |
5 | return 0; |
6 | dfs(coins,amount); |
7 | return res==Integer.MAX_VALUE?-1:res; |
8 | } |
9 | public void dfs(int [] coins,int amount ){ |
10 | if(amount<0) |
11 | return; |
12 | if(amount==0){ |
13 | res = count==0?res: Math.min(res,count); |
14 | return; |
15 | } |
16 | for(int i=0;i<coins.length;i++){ |
17 | count++; |
18 | dfs(coins,amount-coins[i]); |
19 | count--; |
20 | } |
21 | } |
1 |
|
2 | public int coinChange(int[] coins, int amount) { |
3 | if (amount == 0) |
4 | return 0; |
5 | return dfs(coins, amount, new int[amount]); |
6 | } |
7 |
|
8 | public int dfs(int[] coins, int amount, int[] dp) { |
9 | if (amount < 0) |
10 | return -1; |
11 | if (amount == 0) |
12 | return 0; |
13 | if (dp[amount - 1] != 0) return dp[amount - 1]; |
14 | int min = Integer.MAX_VALUE; |
15 | for (int i = 0; i < coins.length; i++) { |
16 | int res = dfs(coins, amount - coins[i], dp); |
17 | if (res >= 0 && res < min) |
18 | min = res + 1; |
19 | } |
20 | dp[amount - 1] = (min == Integer.MAX_VALUE ? -1 : min); |
21 | return dp[amount - 1]; |
22 | } |
1 |
|
2 | public int coinChange(int[] coins, int amount) { |
3 | if(amount==0) |
4 | return 0; |
5 | int [] dp =new int[amount+1]; |
6 | dp[0] =0; |
7 | for(int i=1;i<=amount;i++){ |
8 | int min =Integer.MAX_VALUE; |
9 | for(int j=0;j<coins.length;j++){ |
10 | if(i-coins[j]>=0&&dp[i-coins[j]]<min) |
11 | min = dp[i-coins[j]]+1; |
12 | } |
13 | dp[i] =min; |
14 | } |
15 | return dp[amount]==Integer.MAX_VALUE?-1:dp[amount]; |
16 | } |
1 |
|
2 |
|
3 | int ans=Integer.MAX_VALUE; |
4 | public int coinChange(int[] coins, int amount) { |
5 | Arrays.sort(coins); |
6 | dfs(coins,coins.length-1,amount,0); |
7 | return ans==Integer.MAX_VALUE?-1:ans; |
8 | } |
9 | public void dfs(int[] coins,int index,int amount,int cnt){ |
10 | if(index<0){ |
11 | return; |
12 | } |
13 | for(int c=amount/coins[index];c>=0;c--){ |
14 | int na=amount-c*coins[index]; |
15 | int ncnt=cnt+c; |
16 | if(na==0){ |
17 | ans=Math.min(ans,ncnt); |
18 | break; |
19 | } |
20 | if(ncnt+1>=ans){ |
21 | break; |
22 | } |
23 | dfs(coins,index-1,na,ncnt); |
24 | } |
25 | } |
26 |
|
Num1071 字符串最大公因子
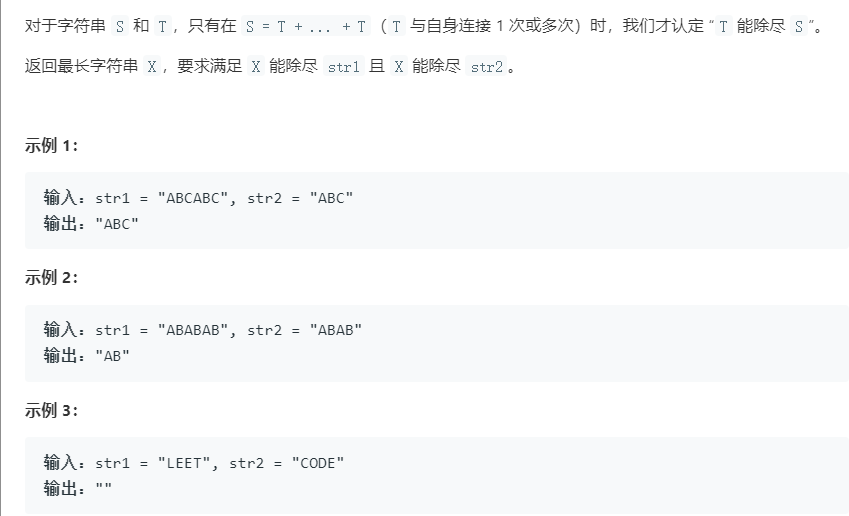
1 |
|
2 | public String gcdOfStrings(String str1, String str2) { |
3 | int len1 = str1.length(),len2 =str2.length(); |
4 | for(int i=Math.min(len1,len2);i>0;i--){ |
5 | if(len1%i==0&&len2%i==0){ |
6 | String temp = str1.substring(0,i); |
7 | if(func(temp,str1)&&func(temp,str2)) |
8 | return temp; |
9 | } |
10 | } |
11 | return ""; |
12 | } |
13 | public boolean func(String res,String str){ |
14 | int len = str.length()/res.length(); |
15 | String s= ""; |
16 | while(len>0){ |
17 | s+=res; |
18 | len--; |
19 | } |
20 | return str.equals(s); |
21 | } |
1 |
|
2 |
|
3 |
|
4 | class Solution { |
5 | public String gcdOfStrings(String str1, String str2) { |
6 | int len1 = str1.length(),len2 =str2.length(); |
7 | int g = gcd(len1,len2); |
8 | if(func(str1.substring(0,g),str1)&&func(str1.substring(0,g),str2)) |
9 | return str1.substring(0,g); |
10 | return ""; |
11 | } |
12 | public int gcd(int a,int b){ |
13 | if(b!=0&&a%b==0) |
14 | return b; |
15 | return gcd(b,a%b); |
16 | } |
1 |
|
2 | public String gcdOfStrings(String str1, String str2) { |
3 | int len1 = str1.length(),len2 =str2.length(); |
4 | if(!(str1+str2).equals(str2+str1)) |
5 | return ""; |
6 | int g = gcd(len1,len2); |
7 | return str1.substring(0,g); |
8 | } |
Num300 最长递增子序列
1 |
|
2 |
|
3 |
|
4 |
|
5 |
|
6 |
|
7 | public int lengthOfLIS(int[] nums) { |
8 | if (nums.length == 0) |
9 | return 0; |
10 | int[] tmp = new int[nums.length]; |
11 | |
12 | |
13 | int res = 0; |
14 | |
15 | for (int num : nums) { |
16 | int left = 0, right = res; |
17 | while (left < right) { |
18 | int mid = left + (right - left) / 2; |
19 | if (num > tmp[mid]) left = mid + 1; |
20 | else right = mid; |
21 | } |
22 | tmp[left] = num; |
23 | if (res == right) |
24 | res++; |
25 | } |
26 | return res; |
27 | } |
Num365 水壶问题
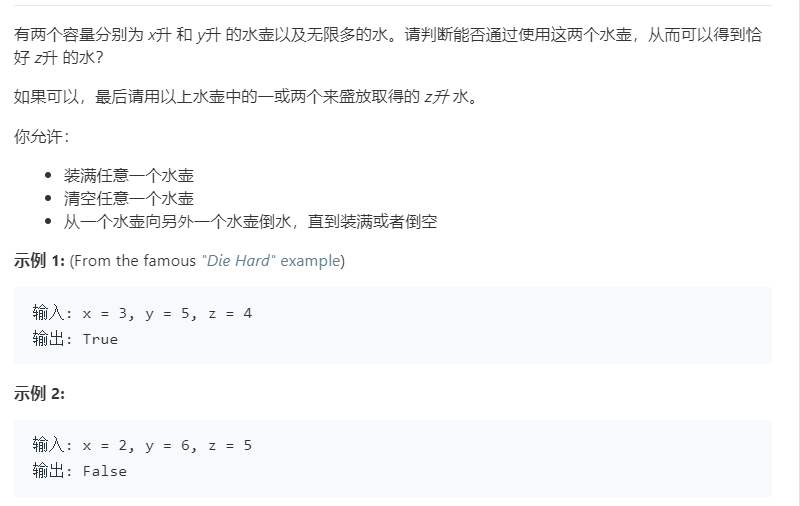
1 |
|
2 | public boolean canMeasureWater(int x, int y, int z) { |
3 | if(z==0) |
4 | return true; |
5 | if(x+y<z) |
6 | return false; |
7 | HashSet<Integer> set =new HashSet<>(); |
8 | Queue<Integer> q = new LinkedList<>(); |
9 | q.offer(0); |
10 | while(!q.isEmpty()){ |
11 | Integer n = q.poll(); |
12 | |
13 | if(n+x<=x+y&&set.add(n+x)) |
14 | q.offer(n+x); |
15 | |
16 | if(n+y<=x+y&&set.add(n+y)) |
17 | q.offer(n+y); |
18 | |
19 | if(n-x>=0&&set.add(n-x)) |
20 | q.offer(n-x); |
21 | |
22 | if(n-y>=0&&set.add(n-y)) |
23 | q.offer(n-y); |
24 | if(set.contains(z)) |
25 | return true; |
26 | } |
27 | return false; |
28 | } |
1 |
|
2 |
|
3 |
|
4 | public boolean canMeasureWater(int x, int y, int z) { |
5 | if(z==0) |
6 | return true; |
7 | if(x+y<z) |
8 | return false; |
9 | return z%gcd(x,y)==0; |
10 | } |
11 | public int gcd(int x,int y){ |
12 | if(y==0||x%y==0) |
13 | return y; |
14 | return gcd(y,x%y); |
15 | } |
Num945 使数组唯一的最小增量
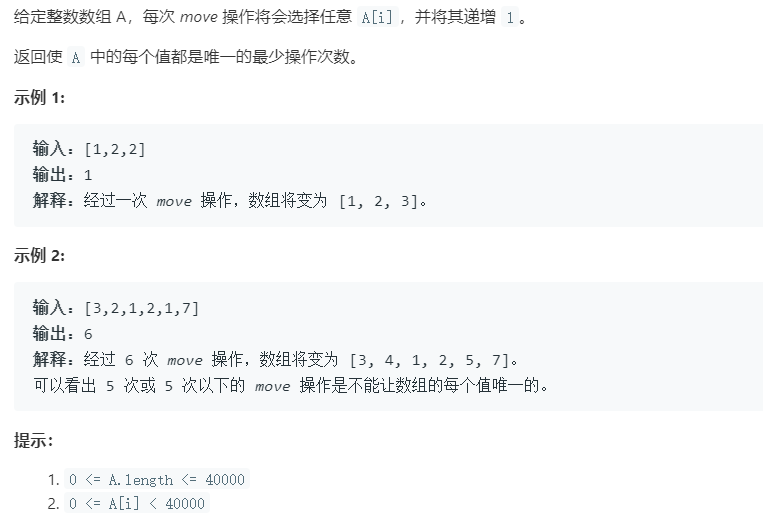
1 |
|
2 |
|
3 | public int minIncrementForUnique(int[] A) { |
4 | if(A.length<=1) |
5 | return 0; |
6 | PriorityQueue<Integer> queue = new PriorityQueue<>(); |
7 | for(int a:A){ |
8 | queue.offer(a); |
9 | } |
10 | int pre =-1; |
11 | int cur =-1; |
12 | int ans =0; |
13 | while(!queue.isEmpty()){ |
14 | cur = queue.poll(); |
15 | if(pre!=-1&&cur<=pre){ |
16 | ans+=(pre-cur)+1; |
17 | cur += (pre-cur)+1; |
18 | } |
19 | pre = cur; |
20 | } |
21 | return ans; |
22 | } |
1 |
|
2 | class Solution { |
3 | int[] pos = new int [80000]; |
4 | public int minIncrementForUnique(int[] A) { |
5 | Arrays.fill(pos, -1); |
6 | int move = 0; |
7 | |
8 | for (int a: A) { |
9 | int b = findPos(a); |
10 | move += b - a; |
11 | } |
12 | return move; |
13 | } |
14 |
|
15 | |
16 | private int findPos(int a) { |
17 | int b = pos[a]; |
18 | |
19 | if (b == -1) { |
20 | pos[a] = a; |
21 | return a; |
22 | } |
23 | |
24 | |
25 | b = findPos(b + 1); |
26 | pos[a] = b; |
27 | return b; |
28 | } |
29 | } |
30 |
|
31 |
|
32 |
|
33 |
|
34 |
|
Num16 按摩师
题目链接:https://leetcode-cn.com/problems/the-masseuse-lcci/
1 |
|
2 | class Solution { |
3 | int res =0; |
4 | public int massage(int[] nums) { |
5 | dfs(nums,0,nums.length,0); |
6 | return res; |
7 | } |
8 | public void dfs(int [] nums,int index,int len,int value){ |
9 | if(index>=len){ |
10 | res =Math.max(res,value); |
11 | return ; |
12 | } |
13 | for(int i=index;i<len;i++) |
14 | dfs(nums,i+2,len,value+nums[i]); |
15 | } |
16 | } |
1 |
|
2 | public int massage(int[] nums) { |
3 | if(nums.length==0) |
4 | return 0; |
5 | if(nums.length==1) |
6 | return nums[0]; |
7 | int [] dp = new int[nums.length]; |
8 | dp[0]=nums[0]; |
9 | dp[1] = Math.max(nums[0],nums[1]); |
10 | for(int i=2;i<nums.length;i++){ |
11 | dp[i] = Math.max(dp[i-2]+nums[i],dp[i-1]); |
12 | } |
13 | return dp[nums.length-1]; |
14 | } |
1 |
|
2 | public int massage(int[] nums) { |
3 | if(nums.length==0) |
4 | return 0; |
5 | if(nums.length==1) |
6 | return nums[0]; |
7 | int tmp_max=0,max=0; |
8 | for(int i=0;i<nums.length;i++){ |
9 | int temp = Math.max(tmp_max+nums[i],max); |
10 | tmp_max = max; |
11 | max = temp; |
12 | } |
13 | return max; |
14 | } |